Creating custom UI elements in Flutter using RenderBox
In Flutter, a RenderBox is a graphical element that has been painted. It has a origin and a paintable area. It is, in essence, what gets drawn on the screen.
A RenderBox is, therefore, a fundamental building block for creating custom user interface (UI) elements in Flutter.
To create a custom RenderBox, you will need to subclass the RenderBox class and override a few of its methods:
paint: This method is called when the render box needs to paint itself. You should use the provided PaintingContext and Offset to paint.
hitTest: This method is called when the user interacts with the app. It should return true if the provided Offset is within the paintable area of the render box.
performLayout: This method is called when the dimensions of the render box change. You should use this method to set the dimensions of the paintable area.
Here is an example of a simple custom RenderBox that draws a red square:
class RedSquareRenderBox extends RenderBox { @override void paint(PaintingContext context, Offset offset) { final Paint paint = Paint(); paint.color = Colors.red; context.canvas.drawRect(offset & size, paint); } @override bool hitTest(HitTestResult result, {Offset position}) { return true; } @override void performLayout() { size = Size(100, 100); } }
To use this RenderBox, you will need to wrap it in a RenderObjectWidget, which can then be used like any other widget in your app.
class RedSquare extends RenderObjectWidget { @override RenderObject createRenderObject(BuildContext context) { return RedSquareRenderBox(); } }
You can then use the RedSquare widget in your app like this:
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return Container( child: RedSquare(), ); } }
I hope this helps! Let me know if you have any questions.
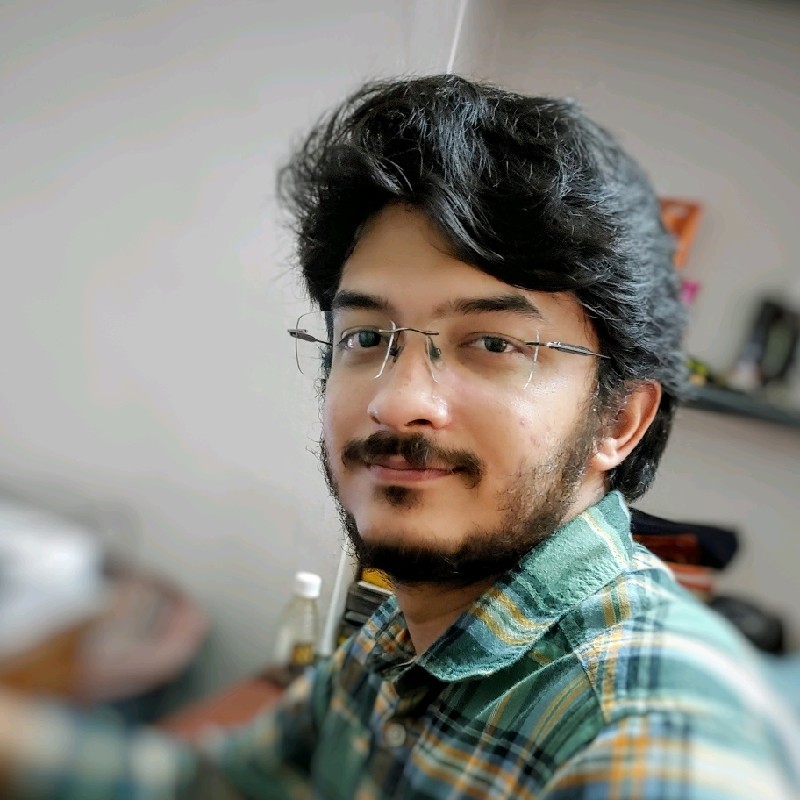
Author: Anil Rao K