Dart - Converting between types | EASIEST Way [2022]
In this post, we'll be exploring Dart's type system and how to convert between types.
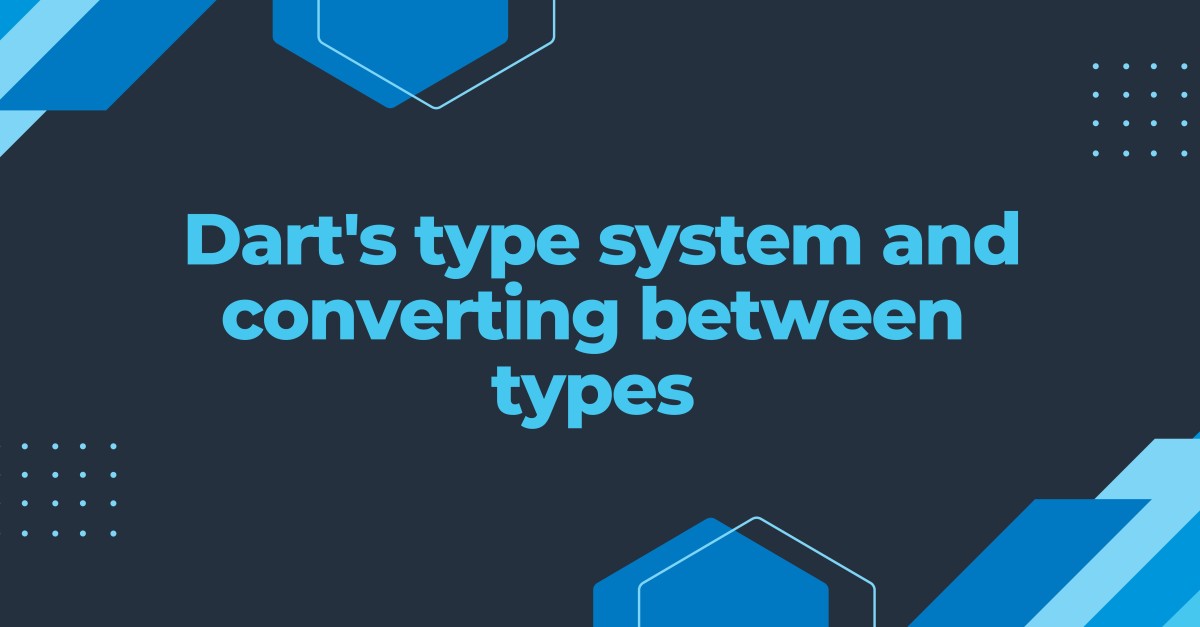
Note:
In this article Flutter and Dart are used interchangeably. Flutter is a framework that uses Dart as its programming language.
So, when we talk about types in Dart, we're talking about types in Flutter.
These are the main topics we'll cover in this article.
- Dart's type system: an overview
- Converting between types in Dart
- The type system in Dart: static vs. dynamic
Dart's type system: an overview
Dart is a statically typed language, which means that every variable and expression has a type.
You can use types to improve the readability and safety of your code.
The Dart type system is sound, meaning that it won’t let you write code that doesn’t make sense.
For example, you can’t assign a variable of type String to a variable of type int.
The type system is also flexible, so you can write code that is easier to read and understand.
For example, you can use the type dynamic to indicate that a variable can hold any type of value.
Dart’s type system is based on type inference.
That means that you don’t have to explicitly specify the type of a variable or expression, the Dart compiler can infer the type from the context.
In general, it is a good idea to let the Dart compiler infer the types of your variables and expressions.
In some cases, however, you might need to explicitly specify a type.
For example, if you are writing a function that takes an int and returns a String, you would need to explicitly specify the types of the parameters and the return value.
The types that are available in the Dart type system are:
1. void
2. dynamic
3. Object
4. num
5. int
6. double
7. String
8. bool
9. List
10. Map
11. Set
12. Iterable
13. Future
Converting between types in Dart
If you've ever used the JavaScript parseInt function, you may be familiar with the concept of parsing strings to convert them into numbers.
The Dart equivalent of parseInt is the int.parse function.
You can use int.parse to convert a string to an integer, and you can use double.parse to convert a string to a double.
If you want to parse a string to a number, you can use the tryParse function.
This function will attempt to parse a string to a number, and if it fails, it will return null.
The toString function is the opposite of parseInt and double.parse. It will take a number and convert it to a string.
If you want to convert a double to int, you can call toInt() function on the double to convert it to an int.
Similarly, if you want to convert into to double, you can call toDouble() function on the int to convert it to a double.
Here are some examples:
// example for try parse var one = int.tryParse('1'); // example: converting int to double int a = 10; double b = a.toDouble(); // example: converting double to int double c = 10.5; int d = c.toInt(); // example: converting string to int String g = "10"; int h = int.parse(g); // example: converting string to double String i = "10.5"; double j = double.parse(i); // example: converting int to string int e = 10; String f = e.toString(); // example: converting double to string double k = 10.5; String l = k.toString();
Static vs Dynamic
When it comes to programming languages, there are two different types of type systems: static and dynamic.
Static type systems check the type of a variable at compile time, while dynamic type systems check the type of a variable at runtime.
Dart is a statically typed language, meaning that the type of a variable is checked at compile time.
This has a few benefits.
First, it means that the type of a variable can be inferred from its initializer.
Second, it allows the compiler to catch type errors early.
However, if you need the flexibility of a dynamic language in dart, you can annotate any variable with 'dynamic'.
While the 'dynamic' type itself is static, it can contain any type at runtime.
This has a few benefits as well.
First, it means that the type of a variable can be changed at runtime.
Second, it allows for more flexibility in how types are used.
So, which type system is better? Static or dynamic?
The answer to this question depends on your preferences and your needs.
If you want the compiler to catch type errors early, then a static type system is probably a better choice.
If you need the flexibility to change the type of a variable at runtime, then a dynamic type system might be a better choice.
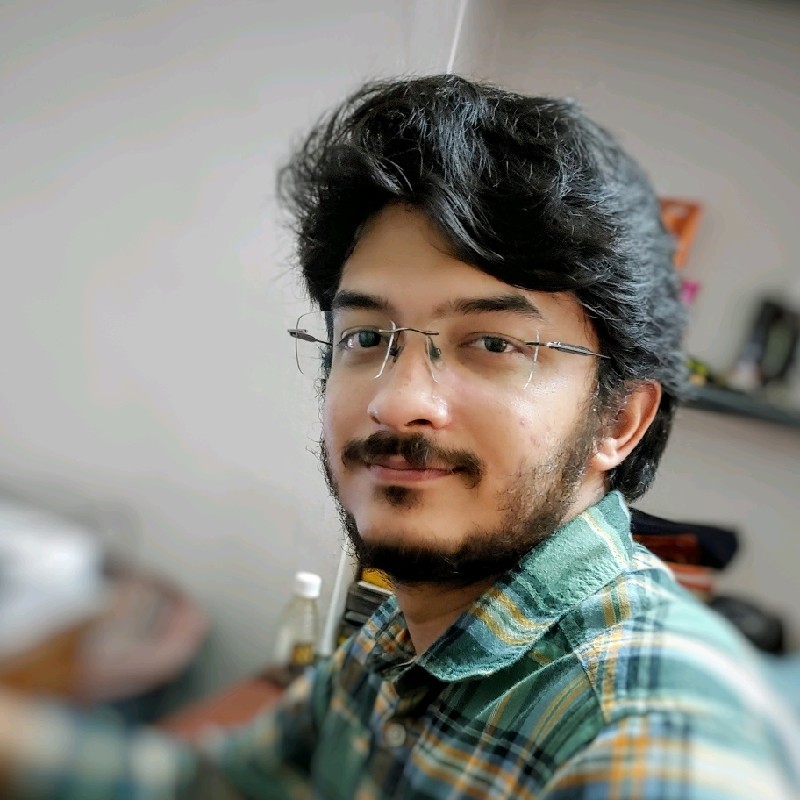
Author: Anil Rao K