Flutter cascade operator (double dots ..) - Ultimate Guide
In Flutter, the cascade operator (..) allows you to make a series of operations on the same object.
This can be useful when you need to perform multiple operations on an object and you want to avoid writing the same code over and over.
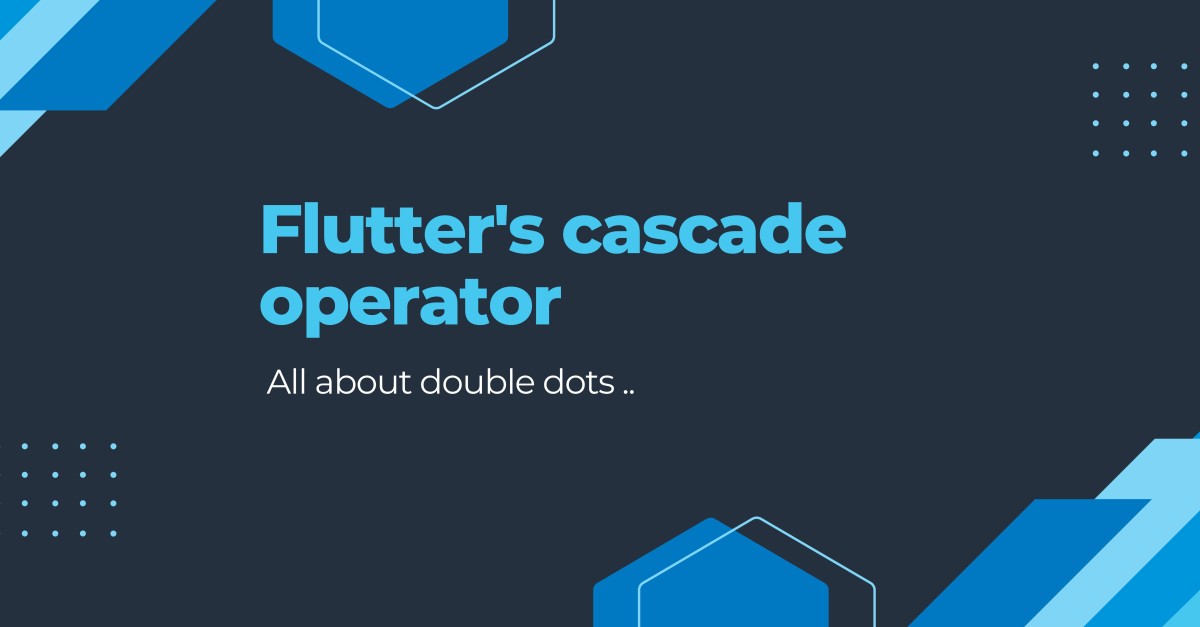
For instance, you can create a new object and initialize it, then use the cascade operator to call methods on that object.
In this way, you can write more concise code.
Let us look at a concrete example. Let us say we have a simple 'Person' class with name & age fields like below.
class Person { String? name; int? age; void increaseAgeBy(int years) { age = (age ?? 0) + years; } void reduceAgeBy(int years) { age = (age ?? 0) - years; } void display() { print('$name is $age years old'); } }
If we wanted to set the name & age to the Person object, and call increaseAgeBy, reduceAgeBy & display methods without the cascade operator, we would code it like below:
var anil = Person(); anil.name = 'Anil'; anil.age = 20; anil.display(); anil.increaseAgeBy(20); anil.display(); anil.reduceAgeBy(10); anil.display();
As you can see we have to point to the 'anil' object instance multiple times to do multiple operations.
This can be simplified with the cascade operator like below:
Person() ..name = 'Anil' ..age = 20 ..display() ..increaseAgeBy(20) ..display() ..reduceAgeBy(10) ..display();
Here we are creating the Person object once and assigning values and calling methods on the same object by simply using the cascade operator.
As you can see, the cascade operator can save you a lot of time and make your code more concise.
The cascade operator can widely be seen in all kinds of classes in the Flutter framework, including widgets, animations, and paints.
There is also an awesome Spread operator in Dart. Click on the following link to learn about the Spread operator and how it simplifies coding in Flutter.
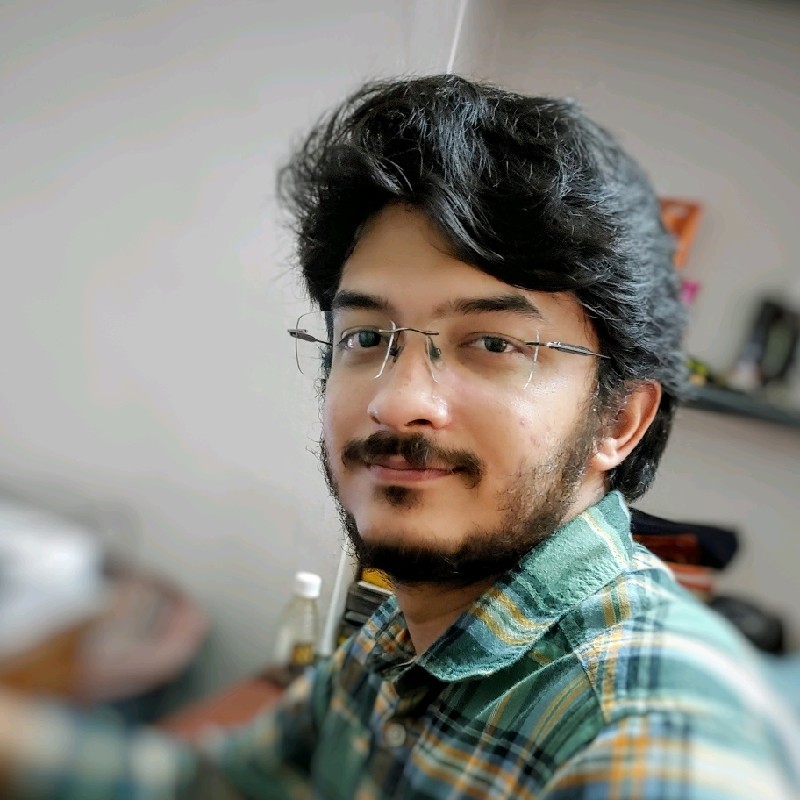
Author: Anil Rao K