Flutter - Stateless Widget
In Flutter, a widget is either a Stateless Widget or a Stateful Widget.
In this article we'll learn about everything on Flutter's StatelessWidget.
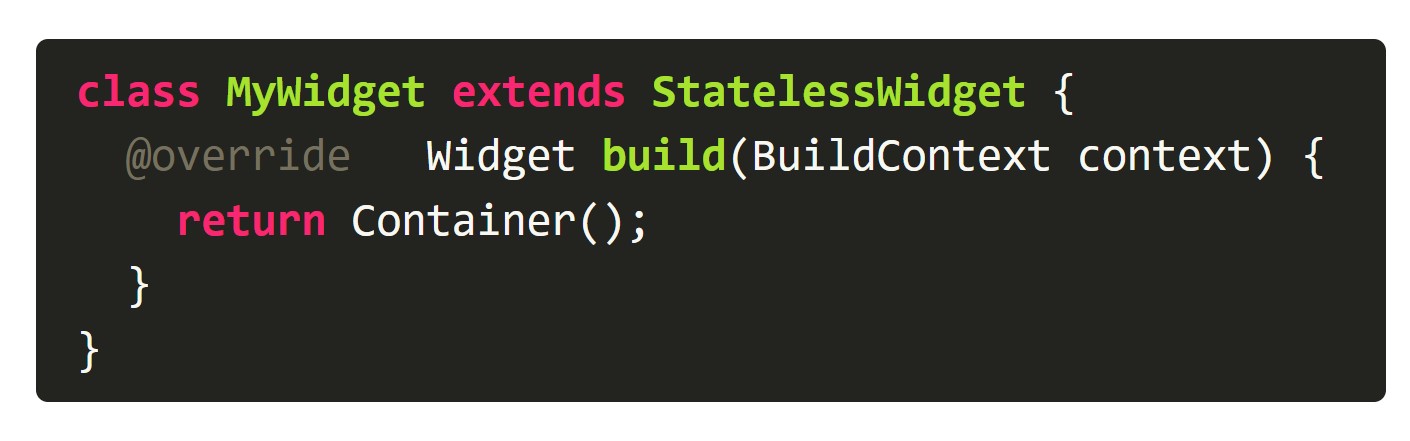
1. What is a Stateless widget?
A Stateless widget is a widget in Flutter that does not keep track of any state information.
In other words, a Stateless widget is a widget that does not have a persistent state.
Stateless widget does not keep track of any information about the current state of the application.
Also, it does not maintain any information about its previous interactions or state.
Instead, Stateless widget simply returns a new widget each time it is called.
This makes Stateless widgets very simple to use and understand.
However, it also means that they are not very flexible.
For example, a Stateless widget might be a static image or a simple text label.
Stateless widgets are created by subclassing the StatelessWidget class.
The build() method is where the widget is actually built, and it is only called once when the Stateless widget is first created.
If you need to keep track of any state information, you will need to use a Stateful widget.
Stateful widgets are rebuilt every time their state changes, and they maintain information about their previous state.
2. How is a Stateless widget different from a stateful widget?
A Stateless widget is a widget that doesn't have a state.
A Stateless widget is a widget that doesn't need to be rebuilt when the state of the app changes.
A Stateful widget is a widget that has a state.
A Stateful widget is a widget that needs to be rebuilt when the state of the app changes.
3. When would you use a Stateless widget?
As discussed, a Stateless widget is a widget that does not require a State object.
Flutter's Stateless widgets are useful when the widget doesn’t need to be rebuilt every time the parent widget changes.
For example, a Stateless widget might be used for a simple button or an icon.
Flutter's Stateless widgets are also useful when the widget doesn’t need to be interactive.
For example, a Stateless widget might be used for a static image or a read-only text field.
4. How do you create a Stateless widget?
You can create a Stateless widget by subclassing the StatelessWidget class.
For example:
class MyWidget extends StatelessWidget { @override Widget build(BuildContext context) { return Container(); } }
Here is a video on YouTube on how to create Flutter's StatelessWidget.
5. What are the benefits of using a stateless widget?
There are numerous benefits to using Flutter's Stateless widgets in your app:
a. Increased Performance:
Stateless widgets are more lightweight and have a smaller memory footprint than their stateful counterparts.
This can lead to improved performance, especially on lower-end devices.
b. Simpler lifecycle:
Stateless widgets have a simpler lifecycle and require less upkeep than stateful widgets.
This can free up valuable time and resources that can be better spent elsewhere in your app.
c. Easier to test:
Because stateless widgets are more predictable in their behavior, they are also easier to test.
This can lead to fewer bugs and a smoother overall development experience.
d. More portable:
Flutter's Stateless widgets are more portable and can be used in more places than stateful widgets.
This makes them ideal for use in libraries and frameworks, or even in other apps.
e. Increased flexibility:
Stateless widgets offer increased flexibility when it comes to layout and design.
This can lead to a more customized and user-friendly experience for your users.
6. Examples of when to use a Stateless widget
When to use a Flutter's Stateless widget:
-If the widget does not need to maintain any state.
-If the widget will never need to update in response to user input.
7. Pros and cons of stateless widgets
If you do not need to remember any information about the user's interaction with your widget, then a Stateless widget is probably a good choice.
This is because Flutter's Stateless widgets are very simple and can be easily rebuilt if necessary.
Stateless widgets are also very easy to test since there is no need to worry about maintaining the state.
However, if you do need to remember information about the user's interaction with your widget, then a Stateless widget is probably not a good choice.
This is because you will need to maintain the state somewhere else, which can be complicated and error-prone.
You have now learned all the basics about Flutter's StatelessWidget.
The next widget you should probably learn about is Flutter's StatefulWidget.
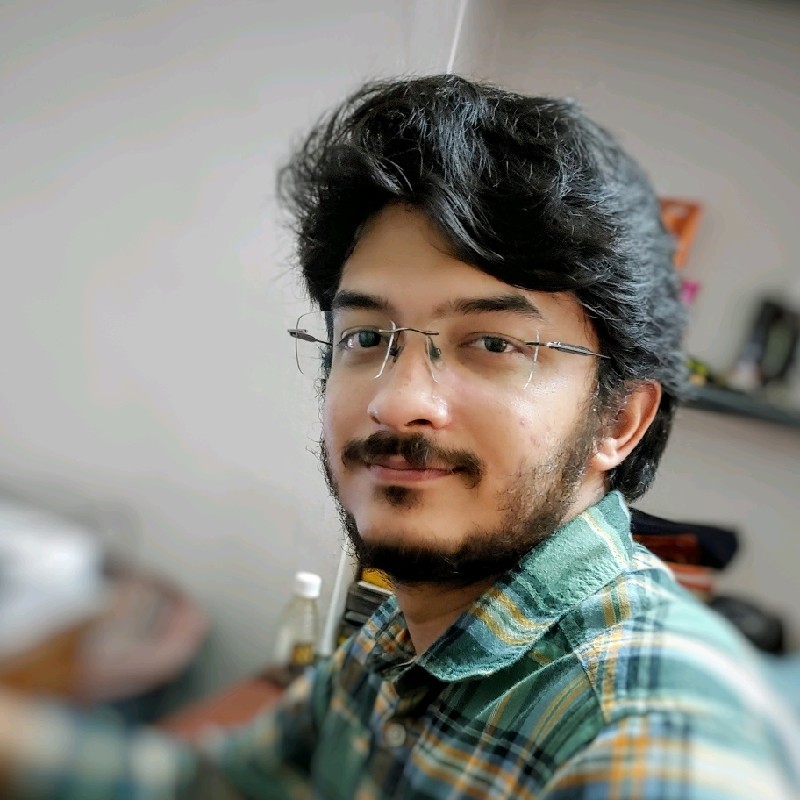
Author: Anil Rao K