Flutter's spread operator (...[]) | Ultimate Guide [2022]
A spread operator can be used to expand an array or collection in Flutter.
This can be useful when you need to add multiple items to a list or when you want to create a new list that is a copy of an existing one.
This operator is also known as the triple dots operator.
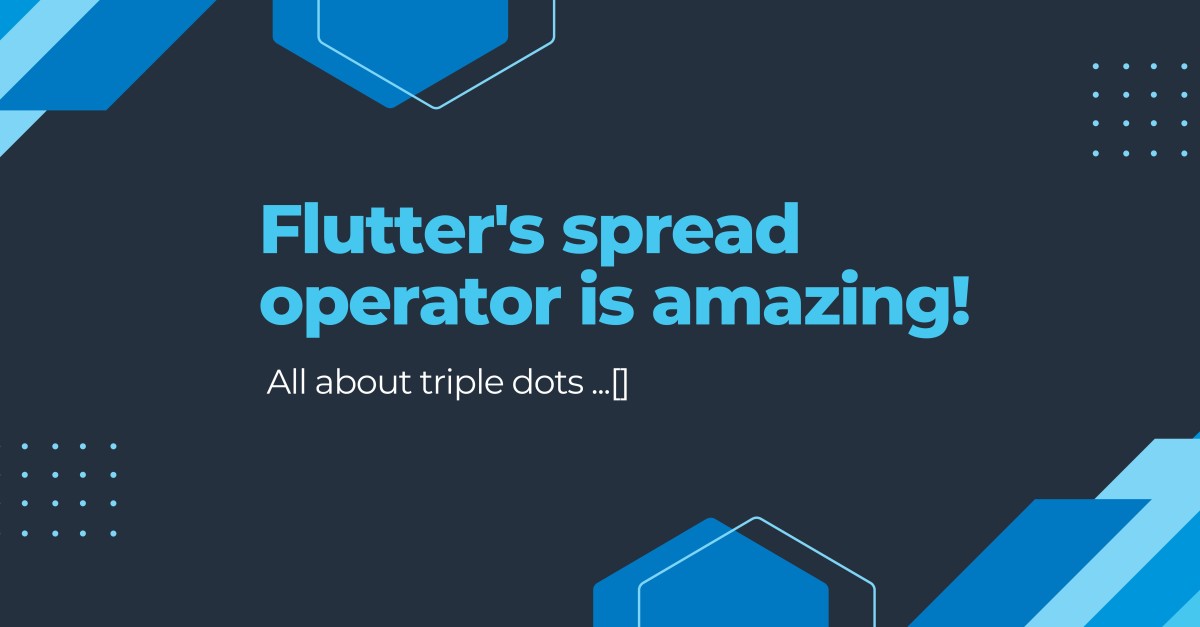
To use a spread operator, you simply place three dots (...) before an array or collection.
This will cause all of the items in the array or collection to be added to the list that you are creating.
Let us look at 4 examples of how to use the spread operator. (4th example is a pro tip specific to Flutter)
1. Spread operator with lists
Creating a new list that is a copy of an existing list
Let's say that you have a list of numbers called myList.
If you wanted to create a new list that contained all of the items in myList, you could use a spread operator like this:
List myNewList = [...myList];
Now, myNewList will contain all of the same items as myList.
2. Creating a new list that contains all the items from multiple lists
Let's say you have a list of numbers:
var numbers = [1, 2, 3];
And you have another list with another list of numbers.
var moreNumbers = [4, 5, 6, 7];
Now if you want to create a new list and want all the numbers from both the list in it, you can use the spread operator:
var allNumbers = [...numbers, ...moreNumbers];
This will add all the items in the numbers list and moreNumbers list to the allNumbers list.
3. Spread operator with maps
The spread operator can also be used with maps.
For example, let's say you have a map of user data:
var user = { "id": 1, "name": "Anil", "age": 30 };
And you want to add another key-value pair to the map. You could create a new map and add the data to it:
var moreData = {"city": "Shivamogga"}; var allData = {...user, ...moreData};
This will add the city key and Shivamogga value to the user map.
4. Using spread operators in Widgets
Let's say we have a page where we want to show the details of a movie to the user.
The details will include the name of the movie, description, reviews, and a write a review button.
Let us assume we want to show all these items one below the other, so we'll consider a Column widget.
Column( children: [ Text('Name'), Text('Example description'), ListView( children: [ // we'll show reviews list here ], ), TextButton( onPressed: () {}, child: Text('Write Review'), ), ], );
Now let us assume our requirement says, that we should show the Reviews list and Write review button only when the user is logged in.
In this case, we can enclose the ListView and TextButton in a list, apply the spread operator and add an if condition to that like below.
Column( children: [ Text('Name'), Text('Example description'), if (isLoggedIn) ...[ ListView( children: [ // we'll show the reviews list here ], ), TextButton( onPressed: () {}, child: Text('Write Review'), ), ], ], );
This way, we can apply conditions on multiple items in an easier manner.
The spread operator is a handy way to add data to lists and maps. It can save you time and make your code more concise.
Note:
In this article Flutter and Dart are used interchangeably. Flutter is a framework that uses Dart as its programming language.
So, when we talk about the spread operator in Flutter, we're talking about the spread operator in Dart.
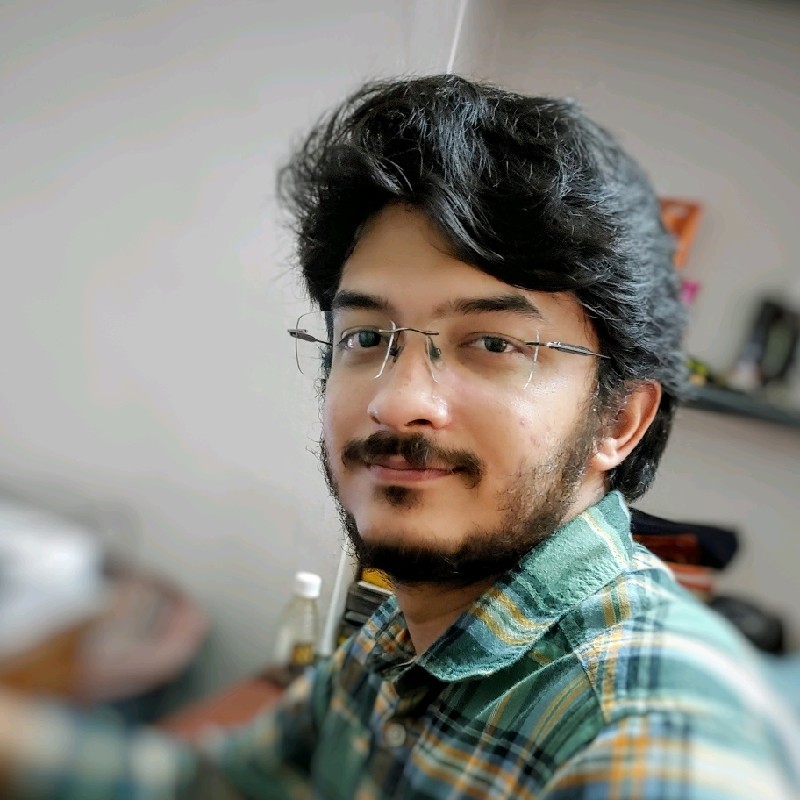
Author: Anil Rao K