How to enable ProGuard?
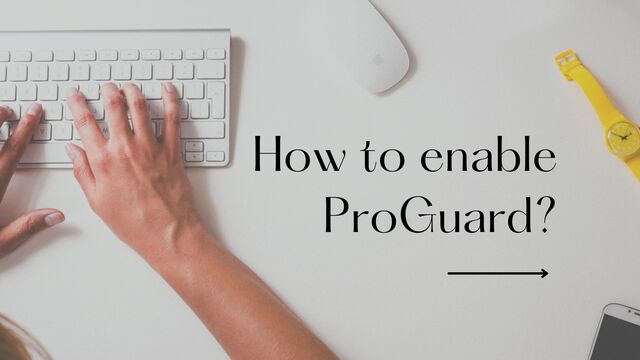
ProGuard is a tool that can be used to shrink, optimize and obfuscate your code, making it harder for attackers to reverse engineer your app.
ProGuard can detect and remove unused classes, fields, methods, and attributes. It optimizes bytecode and removes unused instructions. ProGuard also renames classes and members, and changes the access modifiers of classes and members.
ProGuard is most commonly used by Android developers to shrink and obfuscate their code. By using ProGuard, developers can reduce the size of their Android applications and make it more difficult to reverse engineer.
Once ProGuard is enabled, your app will be built with code obfuscation and shrinking enabled. This can lead to a smaller app size and improved security.
ProGuard rules are usually located in a file called proguard-rules.pro.
This ProGuard rules file is typically located in the root directory of your project.
Here's how to enable ProGuard in your Android project:
1. Create proguard-rules.pro file
Create a file named proguard-rules.pro in your project's root directory and add the classes and their member you want to keep (i.e. everything that ProGuard shouldn't optimize or remove).
Here are a few examples:
Example 1:
-keep public class * { public static void main(java.lang.String[]); } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet); } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet, int); } -keepclassmembers class * extends android.app.Activity { public void *(android.view.View); } -keepclassmembers enum * { public static **[] values(); public static ** valueOf(java.lang.String); } -keep class * implements android.os.Parcelable { public static final android.os.Parcelable$Creator *; }
The proguard-rules.pro file is used to keep certain class files and members from being obfuscated.
The first rule keeps all public classes with a main method from being obfuscated.
The second and third rules keep all classes with public constructors from being obfuscated.
The fourth rule keeps all class members that extend the Activity class from being obfuscated. The fifth rule keeps all enum values and members from being obfuscated. The sixth and final rule keeps all classes that implement the Parcelable interface from being obfuscated.
Example 2:
-dontwarn com.google.android.gms.** -keep class com.google.android.gms.** { *; } -keep interface com.google.android.gms.** { *; }
The first line tells ProGuard not to warn about any unused classes in the com.google.android.gms package.
The second line keeps all classes in the com.google.android.gms package.
The third line keeps all interfaces in the com.google.android.gms package.
Example 3:
-keepattributes *Annotation* -keep class com.google.gson.** { *; } -keep interface com.google.gson.** { *; }
In this example, the rules tell Proguard to keep all annotations, and to keep all classes and interfaces in the com.google.gson package.
Example 4:
-keepattributes *Annotation* -keepclasseswithmembers class * { public static void main(java.lang.String[]); } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet); } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet, int); }
In this case, the file specifies that annotations should be kept, as well as any class with a main method or constructors that take a Context and AttributeSet as parameters.
Example 5:
-keep public class * { public static void main(java.lang.String[]); }
This rule keeps any public class with a main method from being obfuscated.
Example 6:
-keepclassmembers class * { @android.webkit.JavascriptInterface <methods>; }
This rule tells Proguard to keep all methods annotated with @JavascriptInterface when processing webkit classes.
This is necessary when using JavascriptInterface with WebViews.
Example 7:
-dontwarn com.google.** -keep class com.google.** { *; } -keep interface com.google.** { *; }
These Proguard rules will prevent any warnings from being generated for classes and interfaces in the com.google package.
Example 8:
-keepclassmembers class * { public static final String INTENT_*; } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet); } -keepclasseswithmembers class * { public <init>(android.content.Context, android.util.AttributeSet, int); } -keepclassmembers enum * { public static **[] values(); public static ** valueOf(java.lang.String); } -keep class * implements android.os.Parcelable { public static final android.os.Parcelable$Creator *; }
The above proguard rules are used to keep certain class members and classes with members intact when the code is run through Proguard. This ensures that the code behaves as expected when Proguard is used to optimize and obfuscate the code.
2. Point buildTypes to ProGuard files
Open your project's build.gradle file and add the following line to the buildTypes section:
release { useProguard true proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' }
The first line enables ProGuard for the release build type.
The second line tells ProGuard to use the default configuration file (proguard-android-optimize.txt) as well as a custom configuration file (proguard-rules.pro).
The default configuration file(proguard-android-optimize.txt) contains a set of rules that are known to work with most Java apps.
The custom configuration file(proguard-rules.pro) is where you will add any rules that are specific to your app.
FYI, there are two default configuration files provided by Android SDK, proguard-android.txt and proguard-android-optimize.txt.
These two files are located in the /tools/proguard folder of the Android SDK.
PS, you can enable ProGuard for all buildTypes including debug. But enabling ProGuard is not advised as the build times increase substantially.
3. Build your app and test
Run your app in release mode to see the effects of ProGuard.
That's all for this article. Hope this was useful.
If you are using Flutter and want to learn why and how to use ProGuard, please read the article ProGuard in Flutter.
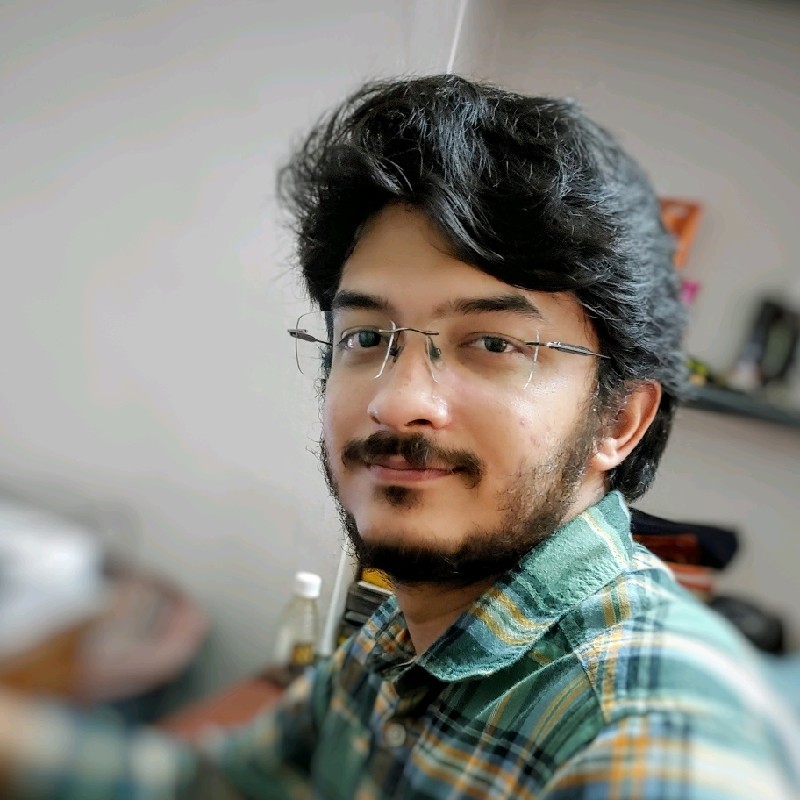
Author: Anil Rao K