How to reduce Flutter app size?
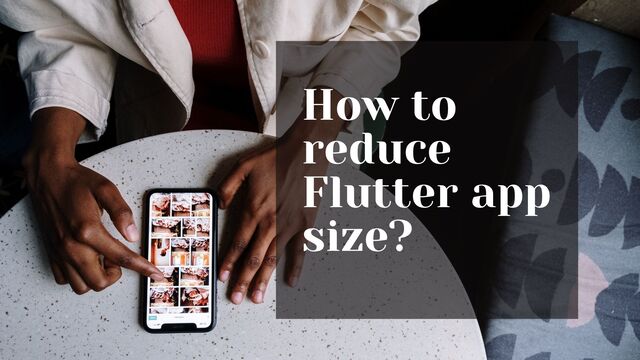
As the world progresses, people are becoming more and more reliant on their mobile devices.
Because of this, developers are always looking for ways to make their apps more user-friendly, and one of the ways they can do this is by reducing the size of their apps.
Smaller app sizes have a number of advantages that can benefit both developers and users.
One of the main advantages of having a small app size is that it can help to improve the speed and performance of the app. This is especially important for users who have slower internet connections or who are using older devices.
Smaller app sizes mean that there is less data to download, which can help to improve the overall speed of the app.
Another advantage of having a small app size is that it can help to save on storage space.
This is important for users who have limited storage on their devices, or who simply want to declutter their device by removing unnecessary apps.
Smaller app sizes can also help to improve the battery life of a device, as there is less data to process when the app is running.
Finally, small app sizes can also benefit developers by making it easier to submit their apps to app stores. This is because many app stores have file size limits, so having a smaller app size can help to ensure that your app can be accepted. In addition, small app sizes can help to improve your app's ranking in app store search results, as they are often sorted by file size.
Overall, there are many advantages to having a small app size. These advantages can benefit both developers and users, and can help to improve the overall experience of using mobile apps.
When you build a Flutter app, the compilation process generates a set of files that are bundled together, similar to how assets are compiled in other mobile apps.
The result is an APK file, which you can distribute to your users.
The size of the APK depends on a number of factors, including the number of features you've included in your app and the size of your app's assets.
However, there are a few ways you can reduce the size of your APK.
1. Use a smaller set of libraries and dependencies
To keep your app's size small, you should use a smaller set of libraries and dependencies. That way, you'll only include the code that you need, and your app will be as lean and efficient as possible.
When choosing libraries and dependencies, look for ones that are lightweight and have few dependencies of their own. That way, you can be sure that they won't bloat your app.
In general, it's best to use the fewest libraries and dependencies possible. But if you do need to use multiple libraries, make sure to carefully consider each one to ensure that it's absolutely necessary.
Remember, every library and dependency you include in your app will add to its size. So, only use the ones that you absolutely need.
2. Minimize the size of your assets
It's no secret that optimizing the size of assets in an app can be a challenge, especially when dealing with complex graphics and multimedia files.
However, there are a few simple tips that can help minimize the size of assets and make your app more efficient.
a. Use vector graphics whenever possible.
Vector graphics are often smaller in size than rasterized images, and they can be scaled up or down without losing quality. This makes them ideal for use in apps, where screen sizes and resolutions can vary widely.
b. When using rasterized images, use the smallest file size possible.
This means using the proper file format (PNG or JPG) and compression settings. For example, PNG files can be further compressed with lossless methods to reduce their size without sacrificing quality.
c. Minimize the use of audio and video files.
These types of assets can be large in size, so using them sparingly can help keep your app's size down.
When possible, use streaming services like YouTube or Spotify instead of including audio or video files directly in your app.
d. Load assets over the network
It is not a good idea to bundle a lot of images with the app. Prefer loading images over the network instead of bundling them with the app.
3. Use App Bundles
Another way to reduce the size of your app is to use App Bundles.
App Bundles allow you to package your app into a single file.
This file can be downloaded by users on demand, which means that they don't have to download the entire app when they only need a small part of it.
To create an App Bundle, you need to use the command "flutter build appbundle".
This will create a file called app.aab in your project's build directory.
You can then upload this file to the Play Store or any other app store.
4. Enable minification and shrinkResources
buildTypes { release { minifyEnabled true shrinkResources true } }
buildTypes are used to customize the way an application is built.
There are two build types by default: release and debug.
The debug build type is used when developing the application and is configured to sign the application with a debug key.
The release build type is used when deploying the application to a production environment and is configured to sign the application with a release key.
The build type can be further customized by adding the following properties:
minifyEnabled: Enables code minification for the release build type.
shrinkResources: Enables resource shrinking for the release build type.
Code minification is the process of removing unused code from the application.
This results in a smaller APK size, which is beneficial for deployments to production environments.
Resource shrinking is the process of removing unused resources from the application.
This also results in a smaller APK size.
Adding the minifyEnabled and shrinkResources properties to the release build type will result in a smaller APK size.
5. Use ProGuard
ProGuard is a tool that can further reduce the size of your Flutter app by removing unused code and resources.
Learn how to use proguard with Flutter in the article "ProGuard in Flutter".
6. Obfuscate
Obfuscate the code to reduce the app size.
Obfuscation is the process of making the code difficult to understand.
This can make the code smaller, as well as make it more difficult for someone to reverse engineer your app.
You can run the below command to obfuscate your code.
flutter build apk --obfuscate --split-debug-info=build/app/outputs/symbols
7. Use google_fonts package
This package allows you to use Google Fonts in your app, which can help to reduce the size of your app by avoiding the need to bundle fonts with your app. It loads the fonts dynamically by making HTTP requests.
To use the google_fonts package, first add it to your pubspec.yaml file:
dependencies: flutter: sdk: flutter google_fonts: ^3.0.1 // or whichever is the latest version
Then, you can use the GoogleFonts.getFont() method to load a font from Google Fonts. For example, to load the Roboto font:
import 'package:flutter/material.dart'; import 'package:google_fonts/google_fonts.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( textTheme: GoogleFonts.robotoTextTheme( Theme.of(context).textTheme, ), ), home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text( 'Hello, world!', ), ), ); } }
This will load the Roboto font from Google Fonts, and use it for the text in your app.
You can also specify a font weight and style when loading a font, which can help to further reduce the size of your app.
By following the tips above, you can minimize the size of your APK and make sure your app is ready for release.
If you are interested, you can learn more about "ProGuard in Flutter" & "How to create a publisher account on pub.dev?" by following the links.
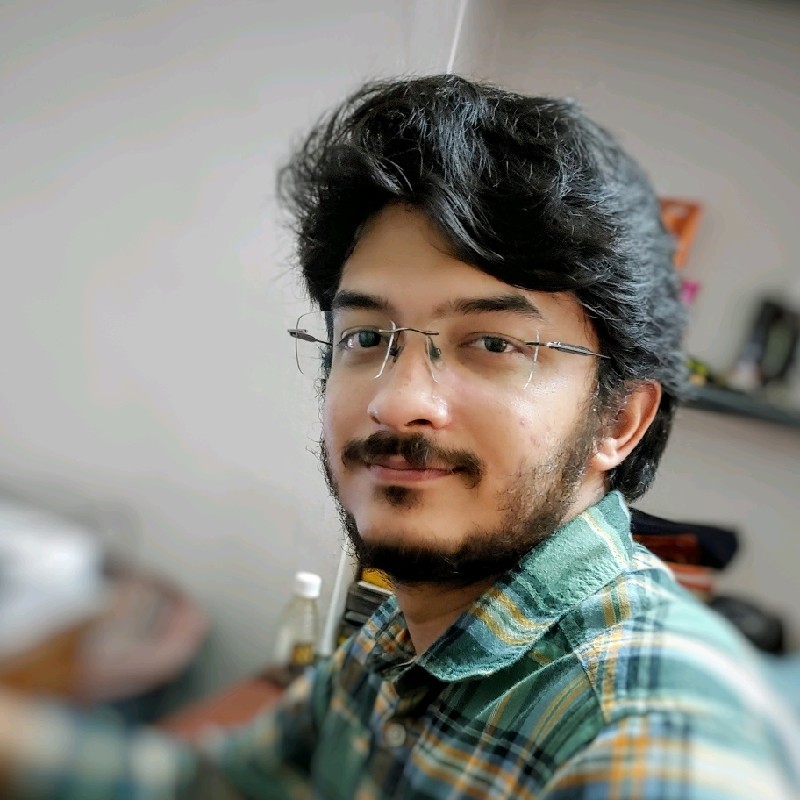
Author: Anil Rao K