Loops in Flutter
Welcome to my blog post on loops in Flutter!
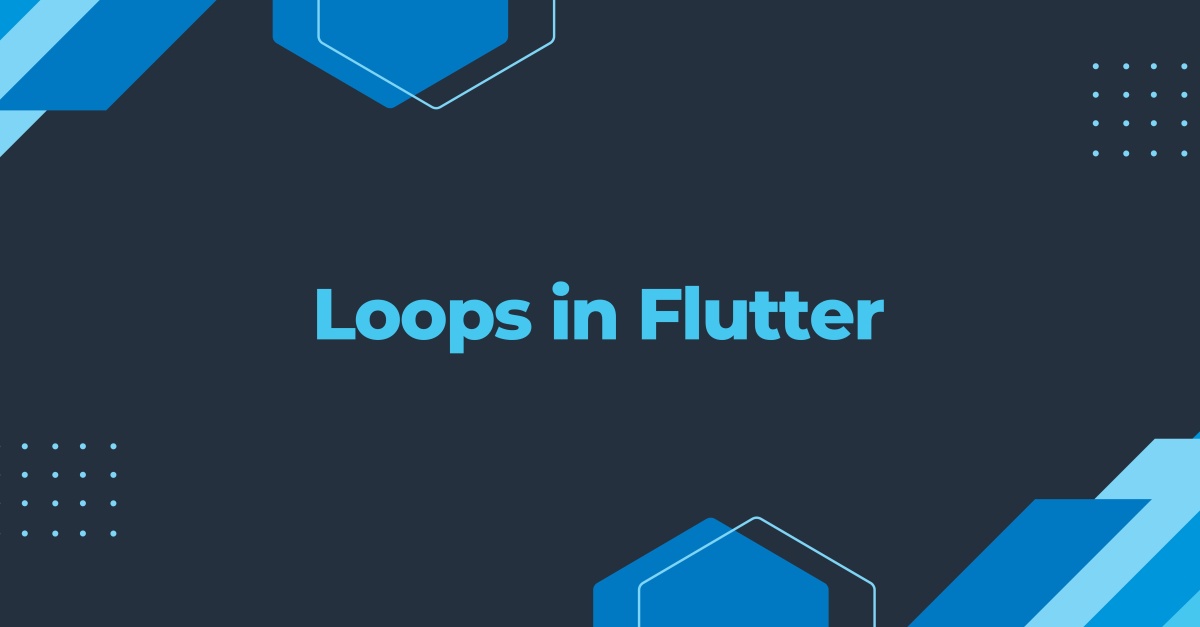
If you're just getting started with Flutter, then you might be wondering what all the fuss is about loops.
In this post, we'll take a look at the different types of loops that are available in Flutter, as well as some of the common ways to use them.
We'll also cover the break and continue statements, which can be used to control the flow of a loop.
Hopefully by the end of this post you'll have a better understanding of how to use these various loops and when each one is appropriate.
Let's get started!
1. What are loops?
Loops are one of the fundamental concepts in programming. A loop is a repeating code block in a program that allows you to iterate through data or perform a certain number of actions.
There are two types of loops: for loops and while loops.
'for' loops are used to iterate through data. The code block in a for loop will execute a certain number of times according to the number you specify as the parameter.
While loops are used to perform a certain number of actions. The code block in a while loop will execute until the condition you specify is no longer true.
Loops are an essential part of programming because they allow you to automate repetitive tasks. For instance, if you wanted to print out the numbers 1 through 10, you could use a for loop to do this.
Loops are also helpful for dealing with data that is stored in arrays.
Arrays are data structures that store a list of values.
By using a for loop, you can iterate through an array and access each value.
If you're new to programming, loops can seem confusing at first. But with a little practice, you'll be able to use them like a pro!
2. What are the different types of loops in Flutter?
Here Flutter and Dart are used interchangeably. Flutter is a framework that uses Dart as its programming language. So, when we talk about loops in Flutter, we're talking about loops in Dart.
There are two main types of loops in Flutter: for loops and while loops.
In 'for' loops, there are three types of loops: for, for-in, and for-each. We'll take a look at each of these in turn.
In 'while' loops, there are two types of loops: while and do-while. We'll also take a look at each of these in turn.
So, in total, there are five different types of loops in Flutter: for, for-in, for-each, while & do-while.
Let's take a look at each of them in more detail.
a. The most common type of loop is the 'for' loop.
A for loop allows you to execute a set of statements a fixed number of times.
The syntax for a for loop is:
for (initialization; condition; increment) { // statements }
In Flutter, the for loop works like this:
for (int i = 0; i < 5; i++) { print('Hello, world!'); }
This code will print "Hello, world!" five times.
The for loop has three parts:
The first part, int i = 0, initializes the loop. The code will start running at i = 0.
The second part, i < 5, is the condition. The code will run as long as the condition is true. In this case, the condition is true as long as i is less than 5.
The third part, i++, is the incrementor.
This is the code that runs after each iteration of the loop. In this case, the incrementor adds 1 to i.
The most common use case for a 'for' loop is to iterate through a list or array.
Here is an example of a for loop that iterates through a list of numbers:
var list = [1, 2, 3, 4, 5]; for (int i = 0; i < list.length; i++) { print(list[i]); }
This code will print each item in the list on a separate line.
b. A for-in loop will execute a set of statements for each element in an array or collection.
The syntax for a for-in loop is:
for (variable in array or collection) { // statements }
In Dart, you can use a for-in loop to iterate over a list, a map, or a set.
When you iterate over a list, you get each item in the list one at a time.
When you iterate over a map, you get each key-value pair one at a time.
When you iterate over a set, you get each value in the set one at a time.
Here’s an example of a for-in loop that iterates over a list:
void main() { var list = [1, 2, 3, 4, 5]; for (var item in list) { print(item); } }
This code prints each item in the list to the console.
Here’s an example of a for-in loop that iterates over a map:
void main() { var map = { 'one': 1, 'two': 2, 'three': 3, }; for (var key in map.keys) { print('Key: $key'); } for (var value in map.values) { print('Value: $value'); } }
This code prints each key and each value in the map to the console.
Here’s an example of a for-in loop that iterates over a set:
void main() { var set = {1, 2, 3, 4, 5}; for (var item in set) { print(item); } }
This code prints each value in the set to the console.
If you want to know the index of each item as you iterate over a collection, you can use the .asMap() method.
This method returns a map where the keys are the indices of the elements in the collection:
void main() { var list = [1, 2, 3, 4, 5]; var map = list.asMap(); for (var key in map.keys) { print('Key: $key'); } for (var value in map.values) { print('Value: $value'); } }
This code prints the index and value of each item in the list to the console.
If you want to iterate over a collection in reverse order, you can use the .reversed property:
void main() { var list = [1, 2, 3, 4, 5]; for (var item in list.reversed) { print(item); } }
This code prints each item in the list to the console in reverse order.
You can use a for-in loop with any iterable object.
c. A forEach loop will execute a function on each element in a collection.
The syntax for a forEach loop is:
collection.forEach((element) => function(element))
The forEach method in Flutter allows you to iterate through a collection and perform an operation on each element.
This is a powerful way to manipulate collections of data.
The forEach method takes a callback function as an argument.
The callback function is invoked for each element in the collection.
The callback function takes one argument, the element.
The element is the current element being processed in the collection.
The forEach method is executed synchronously.
This means that the callback function is called for each element in the collection before the next line of code is executed.
Example:
List list = [1, 2, 3, 4, 5]; list.forEach((element) { print(element); });
This code prints each item in the list to the console.
d. A while loop will execute a set of statements as long as the condition is true.
The syntax for a while loop is:
while (condition) { // statements }
For example, let’s say we want to print out the numbers 1 to 10.
We can use a while loop like this:
int i = 1; while (i <= 10) { print(i); i++; }
This code will print out the numbers 1 to 10 on separate lines.
Let’s break down how it works.
First, we initialize a variable i with the value 1.
This is the variable that we’ll use to track the number that we’re currently on.
Next, we have the while loop.
The code inside the curly braces will execute as long as the condition in the parentheses is true.
In this case, the condition is i <= 10, which means “while i is less than or equal to 10”.
Inside the while loop, we print out the value of i, and then we increment i by 1.
This is important because if we didn’t increment i, the condition would always be true (since 1 is always less than 10), and the code would run forever!
Once i is incremented to 11, the condition is no longer true, and the while loop stops executing.
'while' loops are a powerful tool, but it’s important to use them carefully.
If you forget to increment the variable, or if the condition is always true, your code will run forever and potentially crash your app.
e. A do-while loop is similar to a while loop, except that the condition is checked at the end of each iteration instead of at the beginning.
This means that the statements in a do-while loop will always be executed at least once.
The syntax for a do-while loop is:
do { // statements } while (condition);
Here is an example of a do-while loop in Dart:
void main() { int i = 0; do { print(i); i++; } while (i < 10); }
In the code above, the code block (the print statement) will run 10 times.
The first time it runs, the value of i is 0. The second time it runs, the value of i is 1. And so on, until the value of i is 9.
The condition in a do-while loop can be any expression that evaluates to a boolean value (true or false).
It's important to note that the code block in a do-while loop will always run at least once, even if the condition is false the first time it is checked.
Here is an example of a do-while loop that counts down from 10 to 1:
void main() { int i = 10; do { print(i); i--; } while (i > 0); }
In the code above, the code block (the print statement) will run 10 times.
The first time it runs, the value of i is 10. The second time it runs, the value of i is 9. And so on, until the value of i is 1.
3. Use of continue; and break; statements in loops in Dart
In some cases, you may want to stop the loop before it reaches the end, or you may want to skip a certain number of iterations.
You can use the break and continue keywords to control the flow of a 'for' loop.
The break keyword causes the loop to exit immediately.
Any code after the break keyword will not be executed.
The continue keyword causes the current iteration of the loop to be skipped.
The loop will continue with the next iteration.
Here is an example of using the break and continue keywords in a for loop:
void main() { for (int i = 0; i < 10; i++) { if (i == 5) { break; } print(i); } print('done'); }
This code will print the numbers 0 through 4, then exit the loop.
The string 'done' will be printed after the loop has finished.
Here is an example of using the continue keyword:
void main() { for (int i = 0; i < 10; i++) { if (i % 2 == 0) { continue; } print(i); } }
This code will print the odd numbers 1 through 9.
4. The curious case of forEach loop and why continue; and break; statements don’t work in it
If you've ever tried to use a `continue;` or `break;` statement inside of a Dart `forEach` loop, you may have noticed that it doesn't work the way you expect it to.
The answer is actually quite simple: forEach is not a loop, it’s a method.
What do I mean by this?
A loop lets you execute a set of instructions multiple times until a certain condition is met.
A method is a function that is executed on a specific object.
'forEach' is a method that is called on an array, and it executes a function for each element in that array.
So when you use a continue or break statement inside a forEach method, you are telling the forEach 'method' to continue or break, not the 'loop'.
If you need to use `continue;` or `break;`, you should use a regular `for` loop instead.
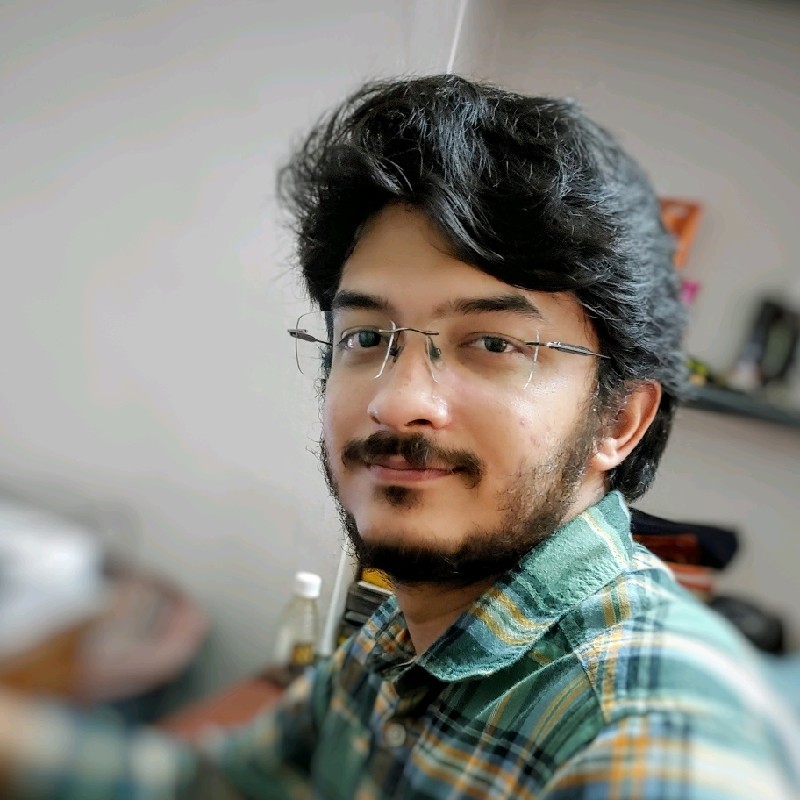
Author: Anil Rao K